. Introduction to Python
Python is a high-level, interpreted language created by Guido van Rossum and released in 1991. It is designed to be easy to read and write, using simple syntax that closely resembles natural language. Python emphasizes code readability and allows developers to express concepts with fewer lines of code compared to languages like C++ or Java.
. Key Features of Python
- Simple Syntax: Python’s syntax is clean and easy to understand, making it an ideal language for beginners.
- Interpreted Language: Python code is executed line-by-line, which makes debugging easier and faster.
- Cross-platform: Python is platform-independent and can run on various operating systems such as Windows, macOS, and Linux.
- Dynamic Typing: Variables in Python don’t need explicit declarations; their types are determined at runtime.
- Extensive Standard Library: Python comes with a large standard library that provides modules and functions for a wide range of tasks.
. Python’s Use Cases
Python is used across many domains due to its flexibility and extensive libraries. Some common use cases include:
- Web Development: Frameworks like Django and Flask allow developers to build robust web applications.
- Data Science and Machine Learning: Libraries like Pandas, NumPy, and TensorFlow make Python a popular choice for data analysis and AI/ML development.
- Automation: Python is often used for automating repetitive tasks such as web scraping, file manipulation, and server management.
- Game Development: Libraries like Pygame make it possible to develop simple games with Python.
- Embedded Systems: MicroPython and CircuitPython bring Python to microcontrollers and IoT devices.
. Python Programming Concepts
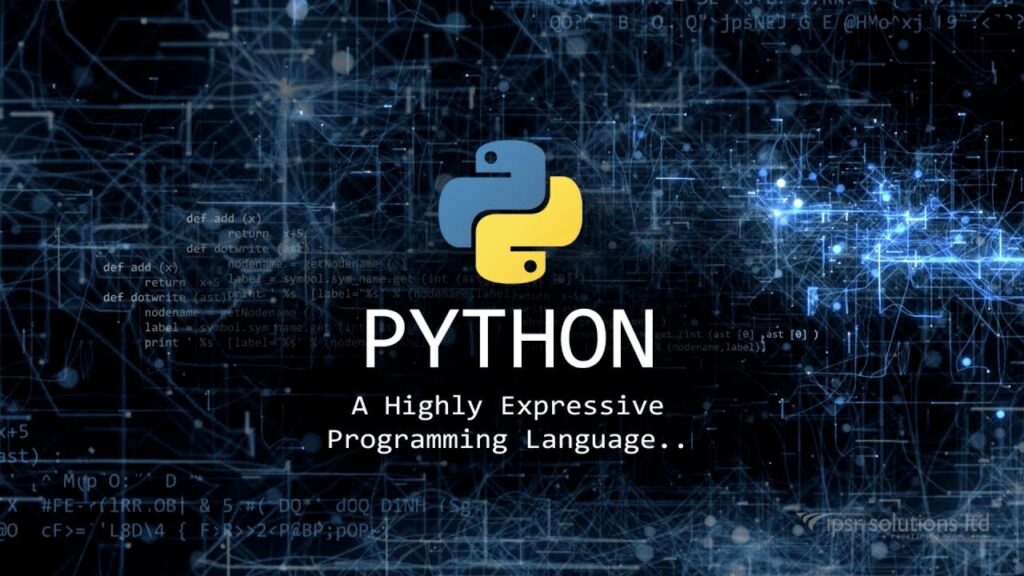
Here are a few fundamental concepts every Python programmer should know:
- Variables and Data Types: Python supports basic data types like integers, floats, strings, and complex numbers, as well as data structures like lists, tuples, dictionaries, and sets.
- Control Structures: Python includes conditional statements (
if
,elif
,else
) and loops (for
,while
) to control the flow of the program. - Functions: Python functions allow you to encapsulate code into reusable blocks. You can define a function using the
def
keyword. - Object-Oriented Programming (OOP): Python supports OOP, allowing you to create classes and objects to model real-world entities.
- Exception Handling: Python provides mechanisms to handle errors gracefully using
try
,except
, andfinally
blocks. - File I/O: Python’s built-in functions and methods make it easy to read from and write to files.
. Python Libraries and Frameworks
Python’s ecosystem is vast, with thousands of libraries and frameworks that extend its capabilities. Some popular ones include:
- NumPy and Pandas: For numerical computations and data manipulation.
- Matplotlib and Seaborn: For data visualization.
- Django and Flask: For web development.
- Scikit-learn: For machine learning.
- TensorFlow and PyTorch: For deep learning and AI.
- Requests: For handling HTTP requests and working with APIs.
. Why Python is So Popular
- Beginner-Friendly: Python’s simplicity and readability make it accessible to new programmers.
- Versatile: Python’s ability to handle a wide range of tasks, from web development to AI, makes it a go-to language for many developers.
- Community Support: Python has a large and active community, which means plenty of resources, tutorials, and third-party libraries are available.
- Industry Adoption: Python is widely used in industry, from startups to large tech companies like Google and Facebook.
. Getting Started with Python
If you’re new to Python, here are a few steps to get you started:
- Install Python: You can download Python from the official website. Make sure to install the latest version.
- Choose an IDE: Integrated Development Environments like PyCharm, VSCode, or Jupyter Notebook can make coding in Python easier and more efficient.
- Learn the Basics: Start with Python basics, including syntax, variables, loops, and functions. Many free tutorials are available online, such as Python’s official documentation.
- Build Projects: The best way to learn Python is by building projects. Start small, then gradually tackle more complex problems.
Conclusion,
Python is a powerful, versatile language that can be used for a wide range of applications. Its simple syntax, large community, and extensive libraries make it an excellent choice for both beginners and professionals. Whether you’re interested in web development, data science, automation, or game development, Python has the tools and frameworks to help you succeed.